What is Link List
W linked list is a data structure consisting of a group of nodes which together represent a sequence. Under the simplest form, each node is composed of a data and a link to the next node in the sequence.
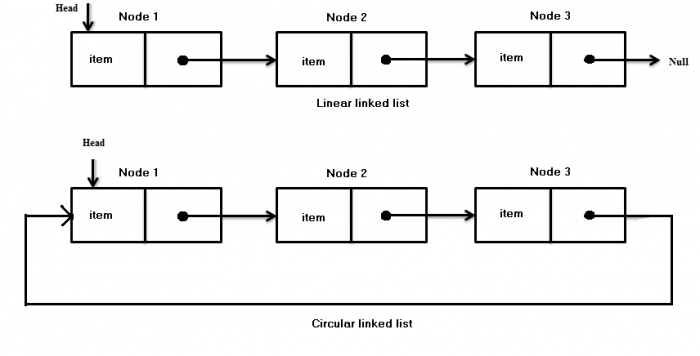
Why Circular Link List
As you can see in the first image that the last->next is null but if last->next is pointing to the head node is called circular link list. The main advantage id that if you have access to any node then you can get access of any node.
Necessary Functions
struct node * create_node(int data)
void insert_at_begining(int info)
void insert_at_position(int info, int position)
void delete_at_begining()
void delete_at_position(int position)
void search(int key)
void update(int olddata, int newdata)
void traverse()
void rev_traverse(struct node *p)
Sample Code
C sample code someone can try C++ code...
struct node
{
int data;
struct node *next;
};
/* We can have additional structure which can have head and last node is node pointer and
additionally can have length variable which contains the length of the link list */
struct node *head = NULL, *last = NULL;
int length = 0;
struct node * create_node(int data)
{
struct node *new;
new = (struct node*)malloc(sizeof(struct node));
new->next = null;
new->data = data;
length++;
return new;
}
void insert_at_begining(int info)
{
struct node *new;
new = create_node(info);
if (head == NULL && last == null)
{
head = last = new;
head->next = last->next = NULL;
}
else
{
new->next = head;
head = new;
last->next = head;
}
}
void insert_at_position(int info, int position)
{
struct node *new, *ptr, *prevnode;
int len = 0, i;
new = create_node(info);
if (head == NULL)
{
if (position == 1)
{
head = last = new;
head->next = last->next = NULL;
}
else
printf("\n empty linked list you cant insert at that particular position");
}
else
{
if (length < position)
printf("\n node cant be inserted as position is exceeding the linkedlist length");
else
{
for (ptr = head, i = 1; i <= length; i++)
{
prevnode = ptr;
ptr = ptr->next;
if (i == position-1)
{
prevnode->next = new;
new->next = ptr;
break;
}
}
}
}
}
void delete_at_begining()
{
struct node *y;
if (head == NULL)
printf("\n List is empty");
else
{
x = last;
y = head;
head = y->next;
last->next = head;
length--;
free(y);
}
}
void delete_at_position(int position)
{
int count = 0, i;
struct node *ptr, *temp, *prevnode;
if (head == last && head == NULL)
printf("\n empty linked list you cant delete");
else
{
if (length < position)
printf("\n node cant be deleted at position as it is exceeding the linkedlist length");
else
{
for (ptr = head,i = 1; i <= length; i++)
{
prevnode = ptr;
ptr = ptr->next;
if (position == 1)
{
length--;
head = ptr;
last->next = head;
free(prevnode);
break;
}
else if (i == position - 1)
{
length--;
prevnode->next = ptr->next;
free(ptr);
break;
}
}
}
}
}
void search(int key)
{
int count = 0, i, f = 0;
struct node *ptr;
if (head == NULL)
printf("\nlist is empty no elemnets in list to search");
else
{
for (ptr = head,i = 0; i < length; i++,ptr = ptr->next)
{
count++;
if (ptr->data == key)
{
printf("\n the value is found at position at %d", count);
f = 1;
}
}
if (f == 0)
printf("\n the value is not found in linkedlist");
}
}
void update(int olddata, int newdata)
{
int i, f = 0;
struct node *ptr;
if (head == NULL)
printf("\n list is empty no elemnts for updation");
else
{
for (ptr = head, i = 0; i < length; ptr = ptr->next,i++)
{
if (ptr->data == olddata)
{
ptr->data = newdata;
printf("value is updated to %d", ptr->data);
f = 1;
}
}
if (f == 0)
printf("\n no such old value to be get updated");
}
}
void traverse()
{
if (head == NULL)
printf("\n List is empty");
else
{
x = head;
while (x->next != head)
{
printf("%d->", x->data);
x = x->next;
}
printf("%d", x->data);
}
}
void rev_traverse(struct node *p)
{
int i = 0;
if (head == NULL)
{
printf("empty linked list");
}
else
{
if (p->next != head)
{
i = p->data;
rev_traverse(p->next);
printf(" %d", i);
}
if (p->next == head)
{
printf(" %d", p->data);
}
}
}