The WPF Expander represents a control with an expanded view where the contents of the expanded area can be expanded or collapsed.
The following XAML code shows how to create an Expander control in WPF.
<Expander Name="ExpanderControl" Header="Click to Expand"
HorizontalAlignment="Left" >
<TextBlock TextWrapping="Wrap" FontSize="14" FontWeight="Light" Foreground="Black">
This is an Expander control. Within this control, all contents will be wrapped.
At run-time, you may expand or collapse this control. Type more text here to be typed.
Jump around and hype.
</TextBlock>
</Expander>
The default view of an Expander control looks like this.

If you click on the header, the expanded view looks like this.

Most of the time, you would want the header of the Expander control to look different from the contents. This can be done by simply setting the font properties of the Expander control different from the contents.
This code sets the FontSize and FontWeight of the Expander control different from the contents of the Expander control.
<Window x:Class="ExpanderControlSample.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Window1" Height="300" Width="300">
<Grid>
<Expander Name="ExpanderControl" Background="LavenderBlush"
HorizontalAlignment="Left" Header="Click to Expand"
ExpandDirection="Down" IsExpanded="False" Width="200"
FontSize="20" FontWeight="Bold" Foreground="Green">
<TextBlock TextWrapping="Wrap" >
This is an Expander control. Within this control, all contents will be wrapped.
At run-time, you may expand or collapse this control. Type more text here to be typed.
Jump around and hype.
</TextBlock>
</Expander>
</Grid>
</Window>
The new output looks like this. As you can see from this figure, the header of the Expander control is different from the contents.

How to create an Expander control dynamically
The Expander class in WPF represents an Expander control.
This code snippet creates an Expander control at run-time.
private void CreateDynamicExpander()
{
Expander dynamicExpander = new Expander();
dynamicExpander.Header = "Dynamic Expander";
dynamicExpander.HorizontalAlignment = HorizontalAlignment.Left;
dynamicExpander.Background = new SolidColorBrush(Colors.Lavender);
dynamicExpander.Width = 250;
dynamicExpander.IsExpanded = false;
dynamicExpander.Content = "This is a dynamic expander";
RootGrid.Children.Add(dynamicExpander);
}
How to set the direction of an Expander Control
The ExpandDirection property of the Expander control sets the direction of the Header. It can be Up, Down, Left or Right. The following code snippet sets the ExpandDirection to Up.
<Expander Name="ExpanderControl" Background="LavenderBlush"
HorizontalAlignment="Left" Header="Click to Expand"
ExpandDirection="Up" IsExpanded="False" Width="200"
FontSize="20" FontWeight="Bold" Foreground="Green">
<TextBlock TextWrapping="Wrap" >
This is an Expander control. Within this control, all contents will be wrapped.
At run-time, you may expand or collapse this control. Type more text here to be typed.
Jump around and hype.
</TextBlock>
</Expander>
The control with Up direction looks like this.

How to set an image in an Expander Header
Expander.Header property may be used to style the header of an Expander control. Within the Header, you can set whatever contents you would like including an Image.
This code adds in image and text in the header of an Expander.
<Expander Name="ExpanderControl"
HorizontalAlignment="Left" Background="LavenderBlush"
ExpandDirection="Down" IsExpanded="False" Width="250"
FontSize="20" FontWeight="Bold" Foreground="Green" >
<Expander.Header>
<BulletDecorator>
<BulletDecorator.Bullet>
<Image Width="50" Source="Flowers.jpg"/>
</BulletDecorator.Bullet>
<TextBlock Margin="20,0,0,0">Flower Header</TextBlock>
</BulletDecorator>
</Expander.Header>
<TextBlock TextWrapping="Wrap" FontSize="14" FontWeight="Light" Foreground="Black">
This is an Expander control. Within this control, all contents will be wrapped.
At run-time, you may expand or collapse this control. Type more text here to be typed.
Jump around and hype.
</TextBlock>
</Expander>
The control with an image header looks like this.

How to add scrolling to an Expander Control
Adding a Scrollviewer control in the contents of an Expander adds scrolling to the Expander. This code adds scrolling to the contents of an Expander.
<Expander Name="ExpanderControl"
HorizontalAlignment="Left" Background="LavenderBlush"
ExpandDirection="Down" IsExpanded="False" Width="250"
FontSize="20" FontWeight="Bold" Foreground="Green" >
<Expander.Header>
<BulletDecorator>
<BulletDecorator.Bullet>
<Image Width="50" Source="Flowers.jpg"/>
</BulletDecorator.Bullet>
<TextBlock Margin="20,0,0,0">Flower Header</TextBlock>
</BulletDecorator>
</Expander.Header>
<Expander.Content>
<ScrollViewer Height="100" VerticalAlignment="Top" >
<TextBlock TextWrapping="Wrap" FontSize="14" FontWeight="Light" Foreground="Black">
This is an Expander control. Within this control, all contents will be wrapped.
At run-time, you may expand or collapse this control. Type more text here to be typed.
Jump around and hype. This is an Expander control. Within this control, all contents will be wrapped.
At run-time, you may expand or collapse this control. Type more text here to be typed.
Jump around and hype.
</TextBlock>
</ScrollViewer>
</Expander.Content>
</Expander>
The Expander control with a scroll viewer looks like this.
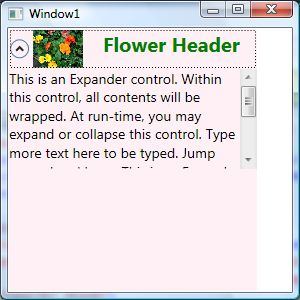