Before Continue to read our first article How to use a TextBlock in WPF and windows phone application Part-1?
http://tech.queryhome.com/94985/how-use-textblock-xaml-wpf-and-windows-phone-application-part
The FontSource property allows loading custom fonts dynamically. The following code snippet sets theFontSource property.
Uri fontUri = new Uri("SomeFont.ttf", UriKind.Relative);
StreamResourceInfo MySRI = Application.GetResourceStream(fontUri);
TextBlock1.FontSource = new FontSource(MySRI.Stream);
Wrapping, Alignment and Padding
The TextWrapping property sets the wrap of no wrap text. The following code snippet sets the wrapping text option.
TextWrapping="Wrap"
The TextAlignment property sets the text alignment in a TextBlock that is of type TextAlignmentenumeration. A text can be aligned left, center, or right.
TextAlignment="Right"
The Padding property sets the space between a boundary and the text that can be applied to all sides or a selected side of the boundary. The padding spacing is based on left, right, top and bottom. If you specify only a single value, the padding will be applied to all four sides and if you specify two values, it will be applied to LeftTop and BottomRight sides.
Listing 5 shows all these properties in a complete sample.
<TextBlock Name="TextBlock1" Height="30" Width="200"
Text="Hello! I am a TextBlock." Foreground="Red"
Margin="10,10,0,0" VerticalAlignment="Top"
HorizontalAlignment="Left"
FontSize="14" FontFamily="Verdana" FontWeight="Bold"
TextWrapping="Wrap" TextAlignment="Center" Padding="2">
</TextBlock>
Listing 5
Inlines
The Inlines property represents the collection of inline text within a TextBlock control. A Run object represents an inline text and can be treated as its own text control and have its foreground and font related properties.
Listing 6 sets the Inlines property of the TextBlock and sets various fonts and foreground colors.
<TextBlock.Inlines>
<Run FontWeight="Bold" FontSize="14" Text="Hi! I am a TextBlock. " />
<Run FontStyle="Italic" Foreground="Red" Text="This is red text. " />
<Run FontStyle="Italic" FontSize="18" Text="Here is some linear gradient text. ">
<Run.Foreground>
<LinearGradientBrush>
<GradientStop Color="Green" Offset="0.0" />
<GradientStop Color="Purple" Offset="0.25" />
<GradientStop Color="Orange" Offset="0.5" />
<GradientStop Color="Blue" Offset="0.75" />
</LinearGradientBrush>
</Run.Foreground>
</Run>
<Run FontStyle="Italic" Foreground="Green" Text="How about adding some green? " />
</TextBlock.Inlines>
Listing 6
The new output looks as in Figure 5.
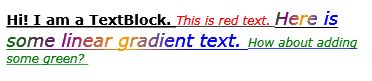
Figure 5
TextDecorations
The TextDecorations property represents the text decorations that are applied to the content of aTextBlock. WPF supports only underlined text decoration.
Listing 7 sets the TextDecorations to underline.
<TextBlock Name="TextBlock1"
Margin="10,10,0,0" VerticalAlignment="Top"
HorizontalAlignment="Left"
FontSize="12" FontFamily="Verdana"
TextWrapping="Wrap" TextAlignment="Left" Padding="2"
TextDecorations="Underline">
Listing 7
The new output looks as in Figure 6.
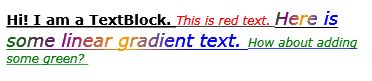
Figure 6
Summary
In this article, I discussed how to create and format a TextBlock control in WPF and C#. Then we saw how to create a TextBlock control dynamically. Then we saw how to set various properties of a TextBlock such as fonts, Inlines and text decorations.