Java Interface
java does not support multiple inheritance. However, there are several cases when it becomes mandatory for an object to inherit properties from multiple classes to avoid redundancy and complexity in code. For this purpose, java provides a workaround in the form of interfaces.
Also, java provides the concept of nested classes to make certain types of programs easy to manage, more secure, and less complex.
Interfaces
An interface in java is a contract that specifies the standards to be followed by the types that implement it. The classes and a class are similar in the following ways:
An interface and a class are similar in the following ways:
- An interface can contain multiple methods.
- An interface is saved with a .java extension and the name of the interface just as a java class.
- Interfaces are stored in packages and the bytecode file is stored in a directory structure that matches the package name.
However, an interface and a class differ in several ways as follows:
- An interface cannot be instantiated.
- An interface cannot have constructors.
- All methods of an interface are implicitly abstract.
- The fields declared in an interface must be both static and final. Interface cannot have instance fields.
- An interface is not extended but implemented by a class.
- An interface can extend multiple interfaces.
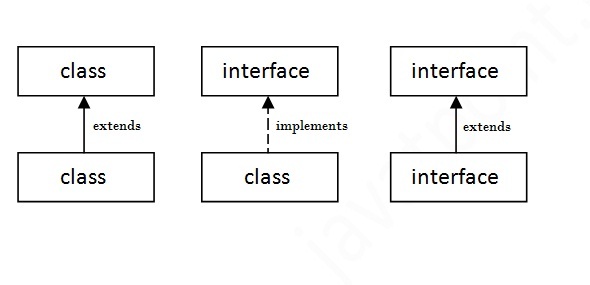
Purpose of Interfaces
Objects in java interact with the outside world with the help of methods exposed by them. Thus, it can be said that, methods serve as the object’s interface with the outside world.
This is similar to the buttons in front of a television set. These buttons acts as an interface between the user and the electrical circuit and wiring on the other side of the plastic casing. When the “power” button is pressed, the television set is turned on and off.
In java, an interface is a collection of related methods without any body. These methods form the contract that the implementing class must agree with. When a class implements an interface, it becomes more formal about the behavior it promises to provide This contract is enforced at build time by the compiler. If a class implements an interface, all the methods declared by that interface must appear in the implementing class for the class to compile successfully.
Thus, in java, an interface is a reference type that is similar to a class. However, it can contain only method signatures, constants, and nested types. There is no method definition but only declaration. Also, unlike a class, interfaces cannot be instantiated and must be implemented by classes or extended by other interfaces in order to use them.
There are a several situations in software engineering when it becomes necessary for different groups of developers to agree to a ‘contract’ that specifies how their software interacts. However, each group should have the liberty to write their code in their desired manner without having the knowledge of how the other groups are writing their code. Java interfaces can be used for defining such contracts.
Interfaces do not belong to any class hierarchy, even though they work in conjunction with classes. Java does not permit multiple inheritance for which interfaces provide an alternative. In Java, a class can inherit from only one class but it can implement multiple interfaces. Therefore, objects of a class can have multiple types such as the type of their own class as well as the types of the interfaces that the class implements. The syntax for declaring an interface is as follows:
Syntax:
<visibility> interface <interface-name> extends <other-interfaces, …>
{
// declare constants
// declare abstract methods
}
Where,
<visibility> : Indicates the access rights to the interface. Visibility of an interface is always public.
<interface-name>: Indicates the name of the interface.
<other-interfaces>: List of interfaces that the current interface inherits from.
For example,
public interface Sample extends Interface1
{
static final int someInteger;
public void someMethod();
}
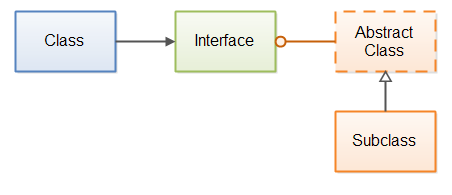
In java, interface names are written in CamelCase, that is, first letter of each word is capitalized, Also, the name of the interface describes an operation that a class can perform. For example,
Interface Enumerable
Interface Comparable
Some programmers prefix the letter ‘l’ with the interface name to distinguish interfaces from classes. For example,
Interface IEnumerable
Interface IComparable
Notice that the method declaration does not have any braces and is terminated with a semicolon. Also, the body of the interface contains only abstract methods and no concrete method. However, since all methods in an interface are implicitly abstract the abstract keyword is not explicitly specified with the method signature.
When a class implements an interface it must implement all its methods. If the class does not implement all its methods, it must be marked abstract. Also, if the class implementing the interface is abstract, one of its subclasses must implement the unimplemented methods. Again, if any of the abstract class subclasses does not implement all the interface methods, the subclass must be marked abstract as well.
The data members of an interface are implicitly static, final, and public.