Introduction
Web services are one of the building blocks of overall .NET architecture. This article explains the basics of ASP.NET web services along with examples.
What are Web Services?
To understand the meaning of term "Web Services" we will take analogous example form COM world. If you are programmer working with Microsoft Technologies, by this time you must have used third party components or at least components developed for your own applications. The COM components you developed provide "services" to your application. For example a component developed for a banking application might be providing services such as loan calculation, interest calculation etc. If you need same business logic at many places such component will be of great use. Components also isolate your business logic from the rest of the application. Web services offer similar functionality. Web services offer "services" over "web". The communication between your application and web service is via HTTP protocol using SOAP standards (Simple Object Access Protocol).
How web services work?
In simple terms it works as follows :
- Your application requests a service (or a method) from a Web Service
- Your request is converted into SOAP format (which is nothing but XML) and routed to web service over web
- The web service processes your request and sends back the result again in SOAP format
For routing your method calls to actual web service a special proxy (which is nothing but a dll) is used. Following figure will make it clear :
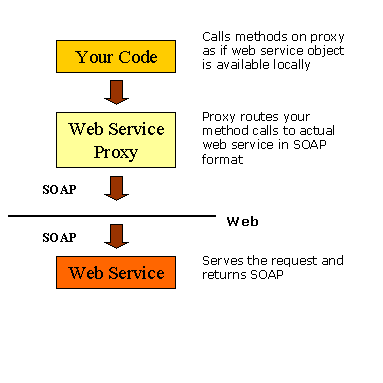
Following points need to be noted about web services :
- Web services are written as plain text files with extention .asmx
- You generate a proxy source code for this .asmx file first into VB or C# source code using a utility provided with .NET (more on that later)
- You then compile the source code into a DLL file (actual proxy dll) which makes the "Web Service" component
- You can consume a web service from any type of application that supports HTTP e.g. Console, WinForms and WebForms or even plain old ASP.
Web Service Description Language
Web Service description language or WSDL is nothing but an XML document describing the web service. It is analogous to Interface Definition Language (IDL) or Type Libraries (TLB). It gives details like service method names, their parameter data types, return values etc.
Creating Your First Web Service
In this section we will create a simple web service called GreetingService. This web service will provide various greeting messages. You will need to pass an integer indicating the type of message you want e.g. Diwali Greetings, New Year Greetings etc. Following are the steps involved in creating a web service :
- Create .asmx file containing source code for the service ( GreetingService.asmx in our case)
- Convert .asmx file into VB or C# source code file
- Compile VB or C# source code thus formed to obtain a proxy dll
- Deploy the resulting DLL to the client applications bin folder
Create GreetingService.asmx file containing source code for the service
<%@ webservice language= "VB" class= "Greetings"%>Imports
System.Web.Services Public
Class Greetings <WebMethod()>Public
Function GetMsg(id as integer) As String
Select case id
case 101
GetMsg="Happy Diwali"
case 102
GetMsg="Happy New Year"
case else
GetMsg="Seasons Greetings"
End Select
End Function
End Class
Let us dissect the code
- Web service files have extention .asmx. In our case we created a file called GreetingService.asmx
- <%@ webservice language="VB" class="Greetings"%>
The webservice directive indicates that this is a web service. Language attribute specifies the language used to write the web service. We have used VB in our example. The class attribute specifies the class name which constitutes the web service. One .asmx file can have multiple class files (may be supporting classes). Out of available classes the class specified in the class attribute is considered for creating web service. - <WebMethod()>public function GetMsg(id as integer) as string
This line marks GetMsg() method as "web callable" method using special attribute <WebMethod()>. This method returns the greeting message based on id we pass.
You can add as many web methods you want to the web service. You can also add normal methods i.e. methods without <WebMethod()> attribute. Such methods will not be exposed over web but can be used internal to the web service. You can also have more than one class per ASMX file. Typically, such classes will act as support class for the main class.
Convert .asmx file into VB or C# source code file
Now that we have our web service source code file ready, we need to convert it into VB source code. To do this .NET comes with a utility called WSDL.EXE. Type in the following command at the DOS prompt :
WSDL http://localhost/mywebapp/GreetingService.asmx?wsdl /l:VB /n:GreetingService
Here,
- http://localhost/mywebapp/GreetingService.asmx?wsdl We need to supply the WSDL of the web service. This can be achieved by appending WSDL to the path of our .asmx file. You can even invoke this URL in browser and view the resulting XML code.
- /l:VB Specifies that the resulting code should be generated in VB
- /n:GreetingService Indicates that name for the resulting namespace should be GreetingService
After executing above command you will get a file called Greetings.vb and it contains the source code for the proxy dll. Do not bother much about the code contained in this file since you will not be generally modifying it manually.
Compile VB or C# source code to obtain proxy DLL
Now we will compile the VB source code to get the proxy DLL.
Type in following Command
vbc
/out:GreetingService.dll
/t:library Greetings.vb
/r:system.dll
/r:system.xml.dll
/r:system.web.services.dll
You will get GreetingService.DLL.
Deploy the resulting DLL
In order to use the DLL you created you must copy it in the \bin folder of your web application.
If such folder is already not there you need to create it
Note : Here, we are assuming that we want to consume our web service from a web client developed in ASP.NET
Creating proxy dll using visual studio.nET
VS.NET provides very easy way to create this proxy dll. It almost hides all the complexities of the process. In your project you can simply right click on references and select "Add a web reference..." . Now select the web service and that's it ! VS.NET will automatically create a proxy for you and place in your web application's bin directory.
Testing our service
You can now test the web service you created by using it in an ASP.NET page. You might use following code :
<%@ language="VB"%>
<script runat=server>
public sub showmsg(s as Object,e as EventArgs)
dim c as GreetingService.Greetings
c=new GreetingService.Greetings()
label1.text=c.GetMsg(101)
end sub
</script>
<form runat=server>
<asp:label id=label1 runat=server text="no msg set yet"/>
<asp:button id=btn1 runat=server onclick="showmsg" text="Click" />
</form>
You can create instances of the web service component just like any other object.
Web services and session state
Just like any ASP.NET application web services can hold session or application state. However, there are some special things to be done.
- Your web service class must derive from WebService. Our class used above is not derived from WebService class and hence can not use session state.
- You need to mark each method that wants to access session state with following attribute :
<WebMethod(EnableSessionState=true)>
The EnableSessionState parameter tells the web service that we want to access session state inside the method.