Introduction
In September 2005 I wrote a five part series on using AJAX with ASP.NET 2.0. The series showed how to use raw AJAX with ASP.NET. Though using raw AJAX did the job of improving responsiveness and reducing post backs, it was certainly considerable effort to achieve the desired result. Now that ASP.NET AJAX is on the anvil things are simple yet powerful. In this article I will give you an introduction to ASP.NET AJAX along with a "hello world" sort of example. In coming weeks and months you will get further installments of the series.
Overview of ASP.NET AJAX
ASP.NET AJAX is the name given to Microsoft's implementation of AJAX. Microsoft already has a sound platform of ASP.NET to develop web site. With the addition of AJAX no doubt it is poised to offer an excellent environment for web site development. Today people look at AJAX for the following main reasons:
- User responsiveness
- Reducing post backs
- Rich user experience
In a traditional web sites when a user submits a form he needs to wait till the complete page is posted back to the server and response is received. That means he needs to wait more. In AJAX the page processing is essentially asynchronous one. Naturally, the web page is more responsive to user actions.
In traditional web applications a web page is frequently posted back to the server even if a small portion of it needs to be changed. This incurs more network overhead and pages take more time to render. In AJAX a small portion of the whole page can be refreshed avoiding any need to post back the complete page. This naturally improves the performance from end user point of view.
ASP.NET provides an impressive array of server controls such as Grids and Lists. However, some UI elements are best rendered using client side script. Menus, short cut menus, progress bars, auto suggestion textboxes, drag and drop, message boxes, modal windows are just some examples. ASP.NET AJAX offers a good range of such controls (more on that later).
Architecture and parts of ASP.NET AJAX
The overall ASP.NET AJAX consists of two main parts :
- Server side components
- Client side components
Note that the word "component" above refers to binaries, scripts and anything else that makes that layer.
Server Side Components
The server side components consists of:
- Server controls
- Web Services
- Server control extensibility
ASP.NET AJAX provides certain server controls that allow you to empower your applications with AJAX functionality. Most notable controls are ScriptManager and UpdatePanel. The ScriptManager control takes care of all the ins and outs of script resources and partial page rendering. On any web form that wants to use ASP.NET AJAX there must be one instance of ScriptManager control. The UpdatePanel control allows you to refresh a part of the whole web form. You can think of it as a control that can turn any web server control into AJAX enabled control.
ASP.NET 2.0 Membership and Profile APIs are part of ASP.NET's server side functionality. Fortunately, ASP.NET AJAX provides a set of web services through which you can consume these features from the client side. For example, from client side script you can check if a user is authenticated and so on.
ASP.NET AJAX also allows you to develop your own AJAX server controls. These controls can have client side "behaviors" and can provide rich and custom functionality as per your requirement.
Client Side Components
ASP.NET AJAX client side components consists of JavaScript script libraries. These script libraries make overall JavaScript more object oriented and add several enhancements to traditional JavaScript. For example, in traditional JavaScript you use document.getElementById() method to access any HTML element on the page. Using AJAX client libraries you can refer to these elements as controls e.g. Label, Selector and Button controls. One good part of ASP.NET AJAX client side components is that they are browser independent. They work on almost all the leading browsers such as IE, FireFox, Safari and others.
NOTE:
Some part of the client side components mentioned above is now separated as "ASP.NET AJAX Futures". Nevertheless, it is part of overall client side components.
ASP.NET AJAX Control Toolkit
ASP.NET AJAX Control Toolkit is a collection of rich AJAX controls that you can use out of the box in your applications. It also provides an SDK for developing your own controls. Some of the controls included in the toolkit are - CollapsiblePanel, ConfirmButton, HoverMenu and ModalPopup.
Developing a Simple AJAX Enabled Application
In order to work through this example you must install ASP.NET AJAX 1.0 RC on your machine. To begin with create a new web site using Visual Studio. Notice that after installing ASP.NET AJAX there is a new web site template named "ASP.NET AJAX Enabled Web Site". The following figure shows the "New Web Site" dialog with this template.
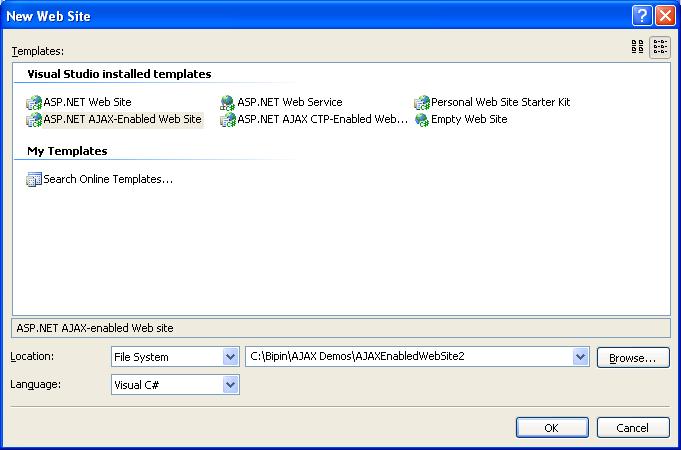
Using this template is not mandetory. However, it adds certain markup in the web.config file. If you don't use this template then you need to do that job on your own. Also, it references System.Web.Extensions assembly. This assembly contains core ASP.NET AJAX functionality.
If you open the default web form you will find a ScriptManager control placed on it. Drag and drop a Label control on the web form. Also drag and drop two UpdatePanel controls and one UpdateProgress control on the web form. Note that these controls are located on the AJAX Extensions node of the toolbox.
Drag and drop a Button and a Label inside the first UpdatePanel control. Drag and drop a Label and a Timer control inside the second UpdatePanel control. Set the Interval property of Timer control to 3000 milliseconds. Finally, drag and drop a Label control inside the UpdateProgress control and set its Text Property to "Wait...Server is processing your request...".
In the Page_Load event of the web form add the following code:
protected void Page_Load(object sender, EventArgs e)
{
Label2.Text = "Time Stamp : " + DateTime.Now.ToString();
}
The code simply sets the Text property of the Label control that is directly placed on the form to current date and time. Now write the following code in the Click event handler of the button that is placed inside the first UpdatePanel.
protected void Button1_Click(object sender, EventArgs e)
{
System.Threading.Thread.Sleep(3000);
Label1.Text = "Time Stamp : " + DateTime.Now.ToString();
}
The code simply sleeps for 3 seconds and then displays current date and time in the Label control. Finally write the following code in the Tick event of the Timer control.
protected void Timer1_Tick(object sender, EventArgs e)
{
Label4.Text = "Timer is ticking : " + DateTime.Now.ToString();
}
The Tick event is raised every time a time span equal to the Interval property is elapsed. The code simply sets the Text of the other Label control to current date and time.
Now set the AssociatedUpdatePanelID property of UpdateProgress control to the ID of first UpdatePanel. The UpdateProcess control is used to display a progress indicator to the end user when the associated UpdatePanel is being refreshed.
That's it! You just developed an AJAX enabled web form. Run the form in the browser and observe the following things:
- When you click on the Button from the first UpdatePanel only the Label inside that UpdatePanel changes its value. The Label on the form remains unchanged. This means only the region of UpdatePanel is getting refreshed.
- When you click on the Button from the first UpdatePanel. the UpdateProgress control shows wait message till the refresh is not complete.
- The label placed inside the second UpdatePanel automatically changes its value after every 3 seconds.
The following Figure shows the web form in action:
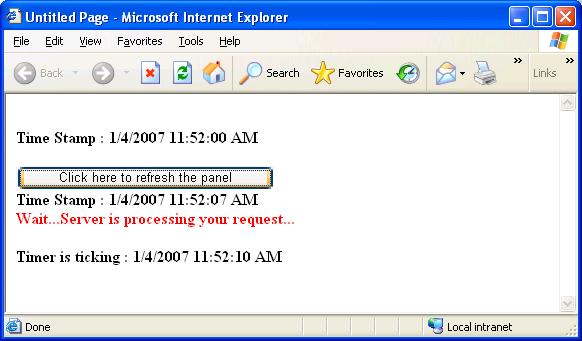