The great thing about XAML is that you can easily change the foreground colour of controls, such as a TextBlock, to be exactly what you want it to be. Whether that is through the built-in colour names or a HEX colour is completely your choice.
However there may be times when you simply wish to change the colour of a control dependent on the value that it is displaying. In this example we will be using a TextBlock that has it's Text value assigned to it using data context binding.
MainPage.xaml
<Page
x:Class="MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:TestProject"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Page.Resources>
<DataTemplate x:Key="listViewItems">
<StackPanel>
<TextBlock
Margin="5,5,5,5"
Text="{Binding Name}"
Style="{StaticResource BaseTextBlockStyle}"/>
<TextBlock
Margin="5,5,5,5"
Text="{Binding Ripeness}"
Style="{StaticResource BaseTextBlockStyle}"/>
</StackPanel>
</DataTemplate>
</Page.Resources>
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<ListView
x:Name="listViewTest"
Margin="5,5,5,5"
VerticalAlignment="Center"
HorizontalAlignment="Center"
ItemsSource="{Binding}"
SelectionMode="None"
IsItemClickEnabled="False"
ItemTemplate="{StaticResource listViewItems}"
ContainerContentChanging="listViewUpdated"></ListView>
<TextBlock
x:Name="listViewNoItems"
Margin="5,5,5,5"
VerticalAlignment="Center"
HorizontalAlignment="Center"
Text="There are no fruits in your list to display!"
Style="{StaticResource BaseTextBlockStyle}"
Visibility="Collapsed"/>
<Button
Width="150"
Height="50"
Margin="20"
VerticalAlignment="Center"
HorizontalAlignment="Right"
Content="Clear fruit"
Click="clearFruitBasket"/>
</Grid>
</Page>
MainPage.xaml.cs
using System.Collections.ObjectModel;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
namespace CSharpCornerTestProject
{
public class Fruit
{
public string Name
{
get;
set;
}
public string Ripeness
{
get;
set;
}
}
public sealed partial class MainPage: Page
{
private ObservableCollection < Fruit > fruitList = new ObservableCollection < Fruit > ();
public MainPage()
{
this.InitializeComponent();
fruitList.Add(new Fruit()
{
Name = "Apple", Ripeness = "Ok"
});
fruitList.Add(new Fruit()
{
Name = "Banana", Ripeness = "Bad"
});
fruitList.Add(new Fruit()
{
Name = "Kiwi", Ripeness = "Rotten"
});
listViewTest.ItemsSource = fruitList;
}
private void listViewUpdated(ListViewBase sender, ContainerContentChangingEventArgs args) {
if (listViewTest.Items.Count == 0)
{
listViewNoItems.Visibility = Visibility.Visible;
listViewTest.Visibility = Visibility.Collapsed;
}
else
{
listViewNoItems.Visibility = Visibility.Collapsed;
listViewTest.Visibility = Visibility.Visible;
}
}
private void clearFruitBasket(object sender, RoutedEventArgs e)
{
// clear the fruit list!
if (fruitList != null) fruitList.Clear();
}
}
}
As I stated above, this is the code base that we used in my previous article about displaying a message once a ListView control no longer has any items in it. We will slightly modify this now so that the ripeness of the fruit in our fruit basket is colour-coded depending on its value.
The first thing we need to do is to create a convertor class for us to use when binding data to the list view. This is a very simple affair and just requires you to add a new "Class file" to your project.
FruitBasketConvertor.cs
using System;
using Windows.UI;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Media;
namespace CSharpCornerTestProject.Convertors
{
public class fruitBasketRipenessForegroundConvertor: IValueConverter
{
public object Convert(object value, Type targetType, object parameter, string language)
{
string ripeness = (value as string);
if (ripeness == "Ok") return new SolidColorBrush(Colors.ForestGreen);
else if (ripeness == "Bad") return new SolidColorBrush(Colors.OrangeRed);
else if (ripeness == "Rotten") return new SolidColorBrush(Colors.DarkRed);
// default return value of lime green
return new SolidColorBrush(Colors.LimeGreen);
}
public object ConvertBack(object value, Type targetType, object parameter, string language)
{
throw new NotImplementedException();
}
}
}
That is all there is to our file, just those 30 lines. Do take note, however, that we have placed the convertors under their own namespace, CSharpCornerTestProject.Convertors.
Using convertors isn't all as daunting as it seems. You create your own custom class, making sure that it derives from IValueConvertor. Then all we need are two functions.
public object Convert(object value, Type targetType, object parameter, string language)
{
// your convertor code goes here
}
public object ConvertBack(object value, Type targetType, object parameter, string language)
{
// your convert back code goes here
}
The Convert function takes the raw value of your binding and allows you to access anything associated with that, whether it is a class, a string, or anything of your choosing.
The ConvertBack function is generally not used all that often and so in our example we are leaving it asthrow new NotImplementedException();. Its main use would be if you wanted to convert the value back to its original state.
Returning to the Convert function, this is very simple and there isn't all that much to it at all. All we do here is take the object value and store it into our string variable, ripeness.
public object Convert(object value, Type targetType, object parameter, string language)
{
string ripeness = (value as string);
if (ripeness == "Ok") return new SolidColorBrush(Colors.ForestGreen);
else if (ripeness == "Bad") return new SolidColorBrush(Colors.OrangeRed);
else if (ripeness == "Rotten") return new SolidColorBrush(Colors.DarkRed);
// default return value of lime green
return new SolidColorBrush(Colors.LimeGreen);
}
Since we have different forms of Ripeness in our Fruit class, we have three separate if statements to determine which colour we should return for our Foreground colour.
When converting a Foreground (or Background) XAML member, it expects a SolidColorBrush to be returned and so that is what we are giving it.
That is everything that we need to do for the code side of things with our convertor, the next step is much simpler and only requires us to add three new lines of code to the existing MainPage.xaml file that we have.
In our Page control, we need to add a new member, highlighted in bold Green.
<Page
x:Class="MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:rTestProject"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:utils="using:TestProject.Convertors"
mc:Ignorable="d">
This will allow us to access the custom convertor class that we just created in FruitBasketConvertor.cs.
Now that we have done that, we need to add our convertor function as a page resource and provide it a key. This will allow us to access it when using the binding convertor. Once again, the addition is highlighted in bold Green.
<Page.Resources>
<utils:fruitBasketRipenessForegroundConvertor x:Key="ripenessConvertor"/>
<DataTemplate x:Key="listViewItems">
<StackPanel>
<TextBlock
Margin="5,5,5,5"
Text="{Binding Name}"
Style="{StaticResource BaseTextBlockStyle}"/>
<TextBlock
Margin="5,5,5,5"
Text="{Binding Ripeness}"
Style="{StaticResource BaseTextBlockStyle}"/>
</StackPanel>
</DataTemplate>
</Page.Resources>
Now that we have given our convertor a key, we can access it anywhere in the MainPage.xaml file when we are binding items. We just have one last thing to do now, that is to add a Foreground member to the TextBlock control that is telling us how ripe our fruit is.
<TextBlock
Foreground="{Binding Ripeness, Converter={StaticResource ripenessConvertor}}"
Margin="5,5,5,5"
Text="{Binding Ripeness}"
Style="{StaticResource BaseTextBlockStyle}"/>
That is everything that we need to do to start dynamically changing the foreground colour of our XAML controls dependent on what the value they're displaying is. If you run the app your fruit basket should now show the Ripeness of each fruit in the three different colours that we assigned earlier on whilst creating our convertor function.
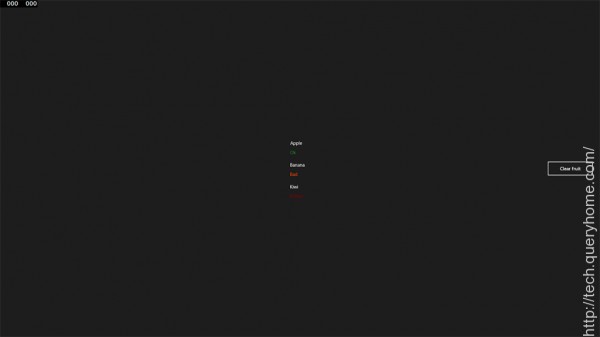